What is the sequence of events that occurs during the execution of a Python related statement as handled by an interpreter?
Any developer can play around with Python code because it has an interpreter. Unlike a compiler, which sits idle until the developer has completed the entire piece of code, the interpreter goes through a language piece by piece and performs it immediately.
The process starts during Lexical Analysis. Whenever a Python program is compiled, the interpreter takes the source code tokens and rends them into an Abstract Syntax Tree which will afterwards be converted into Byte code to be later deployed to the Python Virtual Machine and executed.
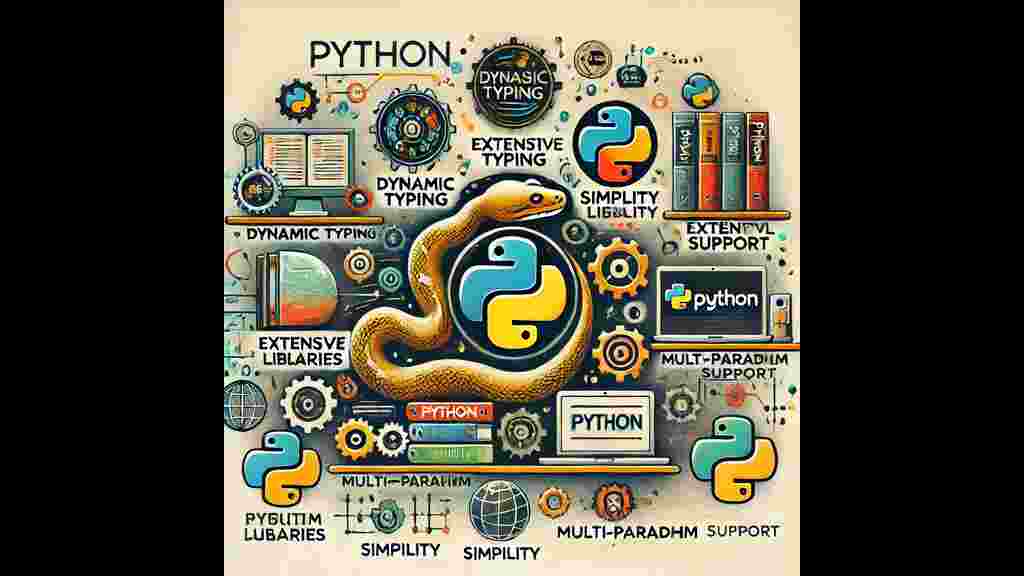
Table of Contents
Python Interpreter
Definition
Python is a computer language which has a high level of abstraction such that it is either interpretative or compiled depending on the user. Due to the vast number of libraries and frameworks offered, it is ideal for web development, data science, machine learning and it is also recommended for first-time programmers since it’s simple and easy to grasp. Interpreters allow programs to be debugged more quickly, although interpreters are slow when compared to compilers.
A token is a small piece of a source program that performs several functions, and it’s what a python interpreter uses to carry out the process of producing a square root of the total bulk of source codes, such as text files and images in a program. The rest of the tokens are further processed by the interpreter which possesses the function of a parser. The parser immediately ends if these tokens witness an illegal state following directives, indentational, colons or parentheses errors leading up to an error displaying message.
After the input has been put through the parser, it results in what is called the Abstract Syntax Tree or the AST in short, which contains the representation of a full Python program. This AST can be compiled down into byte code where in PVM this byte code will finally be used to execute the instructions.
PVM, which stands for Python Virtual Machine, is implemented by performing stack-based manipulation of program data by push and pop operations, executing the program’s byte code instructions that have been compiled by the appropriate translator, maintaining memory and state variables by performing changes when required, dealing with forced errors after incorrect behaviors and the python interactive interpreter command ‘python -c command arg’.
Python variables give us the option of saving values in them which lowers the overall complexity. Their contents may include most simple types like integers and strings, or more complex kinds like lists and dictionaries sorting out your code into simpler parts and easing the reading of the code itself.
‘Type’ with ‘s’ in the end means the ‘kind’ of variables which is their form present in stored variables; in python there are 4 general basic types : intergers, real numbers, letters with meaning and boolean values. While ‘settling’ values to variables using python interpreter scripts, python state these values provisionally to check if such value such an assignment exists. Here x equals int and y carries string value.
When a variable has not been accessed for a while, the interpreter regains the memory occupied by the variable, and this process is called garbage collection, which might cause a loss in the running speed of your program.
In the same breath, however, it should also be considered that while creating any variable in the programming language of Python, the variable names must begin with any letter or underscore, and they have to be unique from other variable names.
Then it is necessary to indicate the type of the variable—for example, if the variable is called, the city must be a string, because ‘city’ is a name that can be set. You can verify this by making a function call, causing the variable to read its content without looking at the codes creating it—for example, in the case when an integer/ float variable is created, and it holds an expression, it evaluates the expression and returns an integer/ float, etc., as result.
Functions
Code interpreters are responsible for transforming code into a format comprehensible to machines through the creation of efficient means of locating variables. And also prevents the medicine from adding or comparing things that do not feet together logically.
After the interpreter converts your program into byte code, it proceeds to execute it from the first line to the last. Although it differs in appearance from the original source code, computers find byte code easier to read and comprehend.
Unique to Python, a function designed with def f (“*args”) or def func(args, val) syntax can work with an undetermined number of positional arguments. This indicates to the Python interpreter that the function can accept in any quantity several positional parameters.
These parameters are incorporated into it and are pushed onto the python call stack when a particular function is to be invoked with its topmost value returning to the stack. If required Python functions can also use the return keyword to indicate that something would be returned when this function completes its task.
Loops in Python programs use a jumping method that is based on one instruction to go to the next one. These allow the conditional statement to jump to the body instructions number nine as the results of the comparison are true for those instructions, while it will go to 34 entirely for those instructions where the false comparison occurs. This will happen in its body in sequence for all the instructions.
Statements
Programmers make statements in their scripts as the core components to carry out any task; every statement has one target action and is a single unit in a program. Statements preside over the program by performing functions such as making numerical calculations or by making decisions; which are key functions in the making of an efficient and effective python programming. Proper usage ensures efficient performance and enables the writing of the cheapest code possible to achieve the results.
Every Python program begins with the process of lexical evaluation, which is the breaking of the source code down to its smallest parts. This source code can be read through and is parsed into words or shapes by an interpreter which is then used to understand what type of expression or statement a particular section or paragraph fall under. For example, If a paragraph contains an equal sign, the following sections will be issued as assignment paragraphs and the ones on the right can be area names, function call areas, and operator areas among other types of areas.
One important difference between expression vs statement is that statement has to provide results whereas expression does not have to do so. Assignments in c language are categorized as statement however python does not classify assignments as expressions. Assignment has a lot to be desired in this language as it relies a lot on compilation that is used to pass the source code into byte code; this difference collapses even worse than if it were in languages like C due to how languages like python do not consider assignment as an expression but instead assignements themselvers have expressions.
As soon as your code gets executed, the interpreter runs and analyses each statement and each block sequentially to see if this particular value can be found in one of the keys in its dictionary and consequently determine what actions should be taken next.
This dictionary has control constructs like an if statement which allows you to skip or only execute a block or numerous statements depending on the result of an if statement; you will learn about other control structures such as looping statements that allow you to perform repetition of a certain block of code repeatedly. In the following tutorials you will learn about more control structures also including looping statements which enable one to repeat parts of code out there over and over again – a very robust prologue to what would eventually determine the rest of programming languages today!
How to resolve failed to pull Helm chart
Expressions
In Python, Expresssion maps as value to the sets of elements that are variables, constants, or symbols of various operations as well as may imply a sequence of function applications. The evaluation of an expression is performed by the python interpreter and the outcome is displayed on the screen- Yet it should be noted that such an order of a number by itself does not foresee receiving a brick on the canvas; for example execution of the statement about assignment, in isolation is not displaying bricks to the screen.
The Python interpreter accepts user-specific inputs with the help of a specialized function known as a parser which studies the inner structure of Python with a context free grammar and checks the correctness of the syntax. These operations done, it gives the simplest meaning of a source statement before its compilation into an executable form which is encapsulated in the acronym:
Compile python program on target computer and run on it also – the user gives a command through the command line or selects from available programs in the window to execute the program; it may run as an independent program or as a component of a web browser program.
A python interpreter is a stack machine hence it also employs stacks while executing python programs. For example, when two numbers are being added in python, first push one onto the stack, then push the other, and when finished with the equation, pop off the stack the first number and print off the result of the equation.