Python Programming Language: A Guide for Beginners
Python is a high-level, interpreted, object-oriented language that has a rich design. It incorporates features of various programming styles including procedural and functional programming as well as object-oriented programming.
For beginners or beginners learning the code language for the first time, Python does have a fairly straightforward and uniform syntax. Its multifunctionality allowed it to gain popularity in the development of web resources as well as in data science and machine learning.
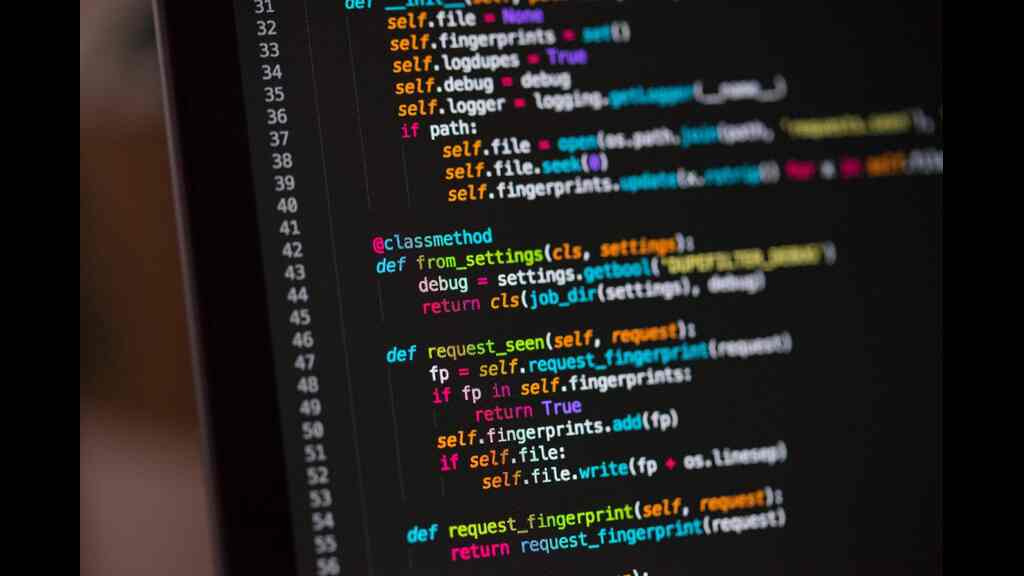
Table of Contents
Python Overview
OOP: Object-Oriented Programming
Object-oriented programming is a pattern of programming that segregates the program logic into multiple objects that has its unique data and actions and can send messages to each object to interact with each other. It also increases maintainability and reusability of code and data.
A class can be defined as a model for an object with instance methods to include & define the behavior, to be easily understood. These instance methods are incorporated in class definitions so as to minimize the exposure of internal information.
Inheritance allows the creation of new classes that share properties and methods with the existing classes. For example, the Penguin class can inherit from the Birds class properties and methods.
Also OOP utilizes polymorphism which refers to the ability of the same object to perform different functions depending on its type. For example, they can be made to execute for a count, or for a certain condition depending on what type of entity it is.
Garbage Collection
Garbage collection is a self-acting process that automatically releases memory otherwise memory leaks would occur while ensuring that objects are disposed off properly. In addition, garbage collection assists improve performance through decreased memory use, while enhancing the stability of programs.
Garbage collector of Python operates by means of reference counting which monitors which of the objects reference each other; when this reference count decreases to zero, those objects do not reference each other anymore and can be reclaimed. In addition to that, this collector uses mark and sweep techniques in order to find cycles in object graphs and uses cycle tracing in this manner too.
Mark and Sweep algorithm starts with a tree structure and traverses each branch until the entire graph is covered while starting from the roots and marking everything that is reachable. Afterwards Sweep takes place where it can locate all the living objects that have not been specifically marked as yet. If during the sweep process an unmarked object is detected, General Clarity examines it and checks whether a cycle surrounds the obejct and sends a call to its finalizers if needed.
Exception Handling
If Python encounters errors that it cannot handle, it comes with the solution of exception and halting the program. This way, the programmers have to do absolutely nothing in order to fix the code or address the error.
Errors that are raised exceptions with values like TypeError, NameError, FileNotFoundError, IndexError, and ValueError types provide information on the cause of the exceptions but can also include user-defined exceptions that are also raised.
Run-time errors in Python programs are handled by try/except blocks. This has become one of its features contributing to its wide adoption in data science and other areas. Moreover, this leads to the acceleration of development processes while facilitating better comprehension of the code with developers. Furthermore, discouragement to learn programming has also been baled out by the wealth of resources including libraries and support of the community.
Interactive Mode
Programmers having the access of the Interactive mode is something unique to python amongst other programming languages, which allows them to test small code snippets or ideas on the go without them having to write the whole program. This mode makes it very easy for developers to stub out code and test out little ideas to see if they will work without having to write an entire program.
Script mode is optimal for writing big projects which require complex logics and structured development, where code is divided into separate functions and modules to aid productivity in writing heavy code. When employing this strategy for tracking error sources in complex projects, the process is made dramatically easier.
For Python scripts, either double-click on a script or use a terminal and enter “python.” It will display a command prompt and ask what to do with it – this can be closed by entering exit() or using the macOS/Linux IDLE program or some other software such as itunes from the window’s taskbar or application menu.
Scalability
Python ranks in the top 5 programming languages in the world along with C, C+, and Java. It’s a language that’s embraced both businesses and individuals alike for machine learning task, software development, web application development, web scraping and automation. However, not every programmer can write a Python program that is infinitely scalable.
Practicing good coding and architecture is a must for developers intending to build extensible and efficient Python apps from scratch. That entails breaking down functionality into smaller reusable functions designed for particular use – this modularizes the code and reduces the maintenance cost as the size and complexity of the program increase.
Always implement strategies that scale applications when the situation requires them. For example, caching helps to lower the load that the server and the database has to support, and database pooling and threading are techniques that assist in proper hardware usage.
How to resolve failed to pull Helm chart
Reusability
Reuse is indispensable In the irreplaceable Principles of Coding Design aspects, it supports the development of maintainable software. Code reuse fosters modular and clean development by eliminating redundancies and allows developers to concentrate on new functionalities instead of rewriting previous ones. However, for reuse to be successful, all the reused code has to be thoroughly documented and tested to ascertain safety and compatibility with other systems.
Python has an effective function which enables one to encapsulate code that is meant to be reused in many occasions. Such blocks of codes are called functions, which are prefixed with the ‘def’ keyword and are invoked on demand. This ensures that there is modularity in programming and helps in reducing duplications.
Functions can also be understood easily because they self-document. The names of parameters also enhance a clarity. This guarantees that the functions will work as designed and will be easy to understand by other developers.