Sentiment Analysis, otherwise known as opinion mining, is a Natural Language Processing(NLP) technique used to classify data as expressing positive, negative or neutral feelings. The technique is quite popular for analysing customer sentiments, social media feedback, product reviews and text based content related to the mood of the people.
Sentiment Analysis looks at the structure of text and identifies the distinct emotional features contained within. For example, for the customer who reviews the product as “The product is awesome! I really love it,” a Sentiment Analyzer would record that as a positive sentiment. On the contrary, something like “I am let down, the product was not what I thought it would be,” would receive a negative tag.
Sentiment Analysis with Default Sentiment Analyzer
Default Sentiment Analyzers Overview
Pre-trained models, known as default sentiment analyzers, are often used in order to make the sentiment analysis tasks easier and faster. These grammars and models are already provided by the libraries NLTK, TextBlob or VADER. These tools are good for the wider range of tasks and also quite easy to implement for fast prototyping or in case someone needs a general analysis of the opinions.
These are some of the default sentiment analyzers that are popular:
NLTK’s VADER Sentiment Analyzer: It is best suited for analyzing sentiments contained within short social media text posts.
TextBlob: It is built for general sentiment and provides a fundamental analysis of interconnected text content sobfor sentiment scoring.
SpaCy: This is a natural language processing library which is a little hard but it possesses important function of allowing building capabilities of sentiment on previously trained language models.
Contribution and Utility of Sentiment Analysis
The contribution of Sentiment Analysis reaches numerous domains:
- Business Intelligence: Product and service enhancement through customer feedback analysis.
- Social Media Monitoring: Negative and hurtful posts on social media platforms are dealt with.
- Brand Management: User’s attraction or dislike about a company’s product on various forums.
- Customer Support: With knowledge of customers feeling, it is easier to attend to customer service.
- By virtue of these applications, organizations are able to achieve meaningful analysis of the reasoning behind their targeted audiences’ attitudes for rational marketing.
Instances and Contexts of Application of Default Sentiment Analyzers
Now, let’s take a few cases in order to clarify, what is a Default Sentiment Analyzer:
Example 1: VADER for Social Media Posts
Let’s say you are trying to figure out what the general public feels about a certain movie using Twitter, right after it’s release, while trying to gauge their overall sentiment.
Text: “It definitely lived up to the expectations!”
Analyzer: VADER (Valence Aware Dictionary and sEntiment Reasoner) is ideal for analyzing social media texts, as underscored by users’ reliance on the use of punctuation, capitalization and emojis.
Analysis: In all possibility, words like “incredible” and “top-notch” would make VADER gauge this event positively with a high score for positivity.
Example 2: Customer Review Sentiment Using TextBlob
Assume a client review which focuses on an online retail product:
Analyzer: TextBlob is generally cited for the analysis of text comments via emphatic scale and assignation of subjectivity.
Analysis: TextBlob would evaluate this review as a negative as it contains such words as “took forever” and “Not happy on the whole”, which show negation.
Example 3: News Sentiment Analysis Using NLTK
Assume that one is analyzing news headlines:
Text “ An unlooked-for fall in the frugality is observed while affectation has constantly been on the upward trend. ”
Analyzer: NLTK (National Language Toolkit) has numerous options for sentiment analysis, but is not optimised for frameworks of social or conversational type.
Analysis: According to NLTK explanation it would most probably evaluate this as negative, when such phrases as “unbearable fall” and “inflation has remained on the growth trend” are still used.
Installing the Libraries that Perform Sentiment Analysis
pip install nltk textblob vaderSentiment spacy
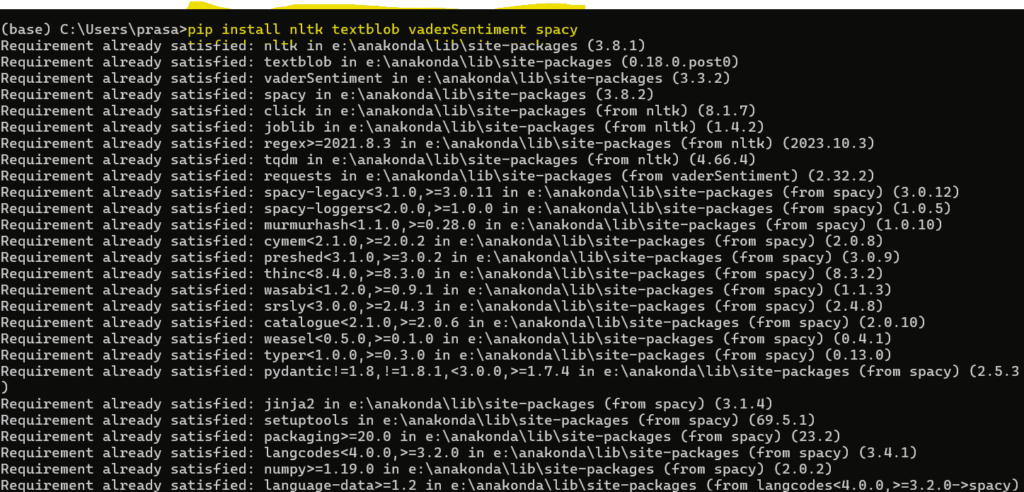
For SpaCy, it is important to have a worked out language model for sentiment analysis. Such as:
python -m spacy download en_core_web_sm
Using a Default Sentiment Analyzer
Detailed Instructions on How to Perform Sentiment Analysis Automatically
In our case, we will perform sentiment analysis in Python with the help of three libraries: TextBlob, VADER, and NLTK. All examples will be provided with the relevant sample code.
Step 1: Make the Necessary Preparations
Make sure that you have the following libraries in your Python environment:
pip install nltk textblob vaderSentiment
Let us engage in an insightful exploration of how to perform sentiment analysis and clarify the objectives of the tasks ahead – analysis of sentiment and its measurement in some given examples.
Example 1: Text Blob to View Product Reviews
#Scenario: Imagine you are in charge of a product on an online store and you intend to read comments and try to understand the sentiments expressed about the product.
from textblob import TextBlob
review = "The phone is amazing! It has a fantastic camera and lasts all day, I could not be happier with my purchase."
blob = TextBlob(review)
# Extracting polarity and subjectivity
polarity = blob.sentiment.polarity
subjectivity = blob.sentiment.subjectivity
print(f"Polarity: {polarity}, Subjectivity: {subjectivity}")
#Output Interpretation: Polarity: 0.5750000000000001, Subjectivity: 0.9
#Subjectivity: Scores close to 1 indicate the review is based on personal opinion rather than fact.
#Explanation: This review will be expected to have a high polarity score because of the sentiments catered in the review like the use of the words such as “amazing,” “fantastic” and “happier,” the customer reflects their satisfaction.
Example 2: Social Media Sentiment Analysis with VADER
#Scenario: Imagine you are a social media manager tracking user sentiment for a new marketing campaign on Twitter.
#Text: "Wow, the new ad campaign is stunning! I love how creative it is. #AmazingAd #MarketingWin"
#Code Implementation:
from vaderSentiment.vaderSentiment import SentimentIntensityAnalyzer
analyzer = SentimentIntensityAnalyzer()
tweet = "Wow, I love how creative it is. #AmazingAd #MarketingWin"
scores = analyzer.polarity_scores(tweet)
print(f"Sentiment Scores: {scores}")
#Compound Score: Scores above 0.05 indicate positive sentiment, scores below -0.05 indicate negative sentiment, and scores close to 0 indicate neutral sentiment.
#Explanation: Words like "stunning" and "love" would boost the positive sentiment score. Hashtags like #AmazingAd and #MarketingWin also increase positivity as they convey excitement.
Example 3: Sentiment Based Customer Service Ticket Classification Using NLTK
#Scenario: Negative sentiment tickets are addressed first in a customer support team's sentiment based matrix.
#Text:: “ I’m so disappointed with the last update of the software. It's bringing up so numerous problems. ”
#Code Implementation:
import nltk
from nltk.sentiment import SentimentIntensityAnalyzer
nltk.download('vader_lexicon')
analyzer = SentimentIntensityAnalyzer()
ticket = "It is throwing so many issues."
scores = analyzer.polarity_scores(ticket)
print(f"Sentiment Scores: {scores}")
#Output Interpretation:
[nltk_data] Downloading package vader_lexicon to
[nltk_data] C:\Users\prasa\AppData\Roaming\nltk_data...
#Negative Score: A high negative score and a low positive score indicate a primarily negative sentiment.
#Explanation: The analyzer would report negativity because of the sentiments 'unhappy' and phrases that suggest 'causing issues.’ This ticket should be given preference for quicker reply.
Improving Sentiment Analysis Results
Default sentiment analyzers serve their purpose, however there are many improvements possible especially where the context and or subtext is not straightforward.
Issues Surrounding Sarcastic Statements
Sarcastic statements may very often be misconstrued especially with default analyzers based on the positive-negative scale. Consider this statement.
Text: “Oh wonderful. Yet another software update that messes everything up.”
The feeling is that of a negative impression even in the face of the word ”wonderful” being present in such a statement. Default sentiment analyzers will see “wonderful” as positive value without appreciating the sarcasm.
Solution: one way to do this is to use automatically trained sentiment models that incorporate aspects of contextual embedding to simplify expression tone and specific word combinations associated with sarcasm.
Performing a Textual Analysis Over a Non-Generic Sociology
A custom lexicon template is useful in enhancing the understanding of alien tongues and thereby makes the relative heartings more axial than the manual norm. For example in the healthcare sector, unique terminologies such as ‘critical’ and ‘positive’ may not generally be positive but have a certain usage context.
For example:
In medicine, test results which are rated “positive” could be detrimental to the patient.
In finance, a certain term that is used is very bearish and if this term is analyzed by general sentiment then hence there is no such notion that can easily be understood by general sentiments.
Utilizing supervised methods and trained labeled data helps in achieving accurate models; these include defined approaches which help provide better analysis of large datasets or industry-specific datasets.
Improving the Standard Models to Fit the Context
Adapting supervised methods and trained labeled data helps in achieving accurate models; these include defined approaches which help provide better analysis of large datasets or industry specific datasets.
Fine-tuning Example:
Gather a dataset related to the end goal (e.g. healthcare reviews, finance articles).
Create clean dataset and tag each value with sentiment.
Train using transfer learning with a model like BERT or a deep learning based model designed specifically for sentiment classification and trained with the ever-increasing number of custom labeled data.
Note:-
If you get any errors please try to run these commands
conda update numpy
conda update h5py
conda install -c conda-forge spacy
python -m spacy download en_core_web_sm
!pip install scikit-learn
conda install -c anaconda scikit-learn
Conclusion
The use of off the shelf sentiment analysis tools is one of the practical ways of performing the sentiment classification of textual data in different domains. NLTK, TextBlob and VADER are libraries that would normally make the task easier and are suitable for the preliminary studies. Not ot be omitted however, as we have seen, default analyzers aren’t without their own failures, in some cases even rendering the context meaningless due to absence of sarcasm, usage of particular jargons, or advanced text.
For better performance in sentiment analysis, especially for Niche areas, it is advisable to use:
Shifting lexicon style
Combining lexicon with machine learning model based style
Modifying the models to be able to address the area of focus based already existing datasets
In short, models employed in either tools or trained specifically for the purpose of opinion mining bring great deal of value to business and research that seeks to explore the patterns in public perception or client views. The method of analysis to be employed determines on the nature of data and specifics of the analysis.